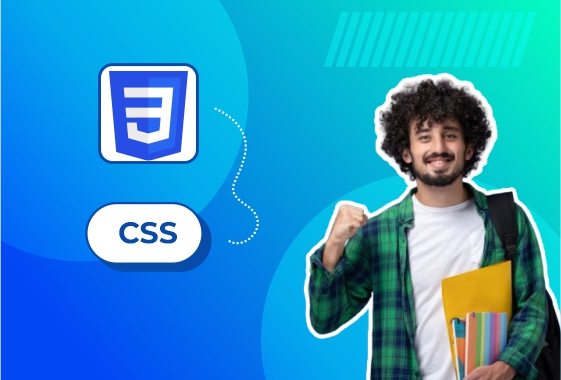
Web Pong Game
Project Title: Web Pong Game (JavaScript Version)
Overview:
The Web Pong Game is a browser-based version of the classic arcade game Pong, built using HTML, CSS, and JavaScript. The game features two paddles and a ball bouncing between them. One paddle is controlled by the player, while the other can be controlled either by a second player or basic AI.
Core Gameplay Features:
- 2 Paddles: One for each side of the screen (player vs player or player vs AI)
- Ball Movement: Ball bounces off the paddles and screen edges
- Collision Detection: Detects ball-paddle and ball-wall collisions
- Scoring System: A point is awarded when a player misses the ball
- Restart Option: Game restarts after each point or game over
Technologies Used:
- HTML5 <canvas>: For drawing the game elements (ball, paddles, text)
- CSS: For styling and positioning the canvas
JavaScript:
- Game loop and animation
- Paddle control with keyboard events
- Ball physics and collision logic
- AI paddle behavior (optional)
How It Works:
- Canvas Setup: The game board is rendered using the HTML5 <canvas> element.
- Drawing Elements: JavaScript draws the paddles, ball, and score on the canvas every frame.
- User Input: Keyboard events (like arrow keys or W/S) move the paddles.
- Ball Physics: The ball moves with a velocity and bounces when hitting a paddle or wall.
- Collision Logic: Detects when the ball hits a paddle or goes out of bounds to update score.
- AI (Optional): A simple AI moves the computer paddle to follow the ball's Y-position.
Learning Objectives:
- Understanding game loops and rendering with requestAnimationFrame()
- Managing keyboard inputs for interactive controls
- Implementing basic physics and collision detection
- Structuring a simple but complete browser game
- Using canvas APIs for 2D rendering
Game Logic Components:
- Ball Object: Handles position, speed, and movement direction
- Paddle Object: Represents both player and AI paddles with position and movement
- Game Loop: Constantly updates game state and redraws the scene
- Score Tracker: Updates and displays score after each goal
- Restart Mechanism: Resets ball and paddles for the next round