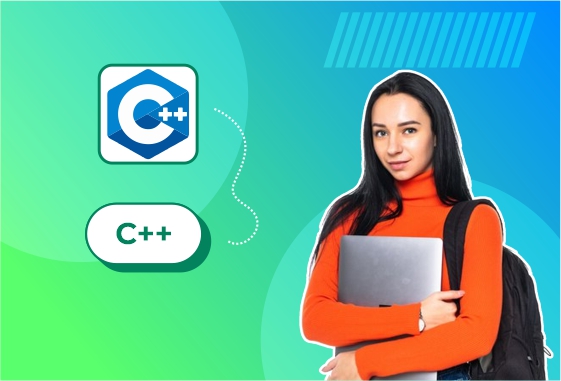
Multiplayer Game with Server-Side Logic
Project Title: Multiplayer Game with Server-Side Logic (C++)
Project Summary
This project is a real-time multiplayer game developed in C++, where players can connect over a network and compete or collaborate. It includes a custom-built server-client architecture, with game logic, synchronization, and data validation handled on the server side. The game can be 2D or 3D, depending on your design (e.g., arena shooter, card game, racing, or platformer).
Core Components
Client-Server Architecture
- Server handles game state, rules, matchmaking, and logic.
- Clients handle user input, rendering, and sending updates to the server.
- Uses TCP/UDP sockets (e.g., using libraries like Boost.Asio or native sockets.h).
Networking Protocol
- Custom protocol for sending and receiving game data (position, actions, events).
- Message serialization/deserialization using C++ structs and byte buffers.
Game Logic on Server
- Validates player moves to prevent cheating.
- Maintains global game state (e.g., player positions, scores, health).
- Broadcasts updates to all connected clients.
Multiplayer Synchronization
- Handles lag compensation and state prediction.
- Server-side interpolation and reconciliation mechanisms.
Authentication and Lobby System
- Players can connect, register, and join lobbies.
- Server manages room creation, player matching, and in-game sessions.
UI and Game Client
Built with a graphics library (e.g., SFML, SDL, or OpenGL).
Real-time UI showing players, scoreboards, and in-game actions.
Error Handling and Security
- Handles dropped connections, timeouts, and invalid packets.
- Prevents cheating by restricting logic to the server side.
Technologies Used
- C++
- Socket programming (TCP/UDP)
- Libraries: Boost.Asio, SFML/SDL/OpenGL (for graphics), CMake
- Multithreading for handling multiple clients
- JSON or binary protocol for data exchange
Learning Outcomes
- Deep understanding of socket programming and networking
- Experience in building client-server systems from scratch
- Real-time synchronization and game state management
- Server-side validation and security concepts
- Efficient C++ architecture for concurrent systems
Possible Enhancements
- Add voice/text chat system
- Implement a ranking system and persistent user profiles
- Use database (like SQLite or MySQL) for storing player stats
- Create a simple mobile or web client version
- Deploy server to cloud (AWS, Azure, or VPS)