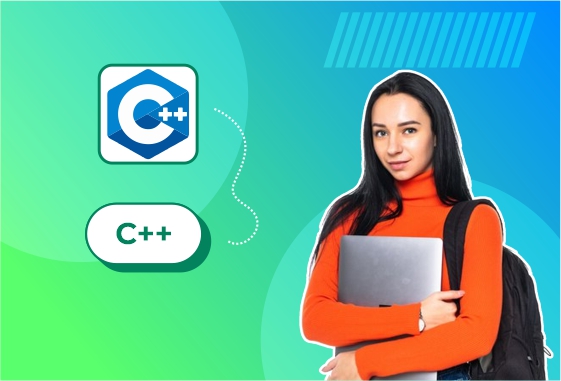
AI Opponent for Chess Game (using C++)
Project Title: AI Opponent for Chess Game using C++
Project Summary
This project involves creating a Chess Game where the player can compete against an AI opponent. The AI opponent is programmed using C++, utilizing algorithms like Minimax and Alpha-Beta Pruning for decision-making, providing a challenging chess experience. The game also includes a graphical user interface (GUI) and can be played locally.
Core Components
Chess Game Logic
- Representation of the chessboard and pieces using arrays or classes (e.g., 8x8 grid, piece objects).
- Legal move generation for each piece type, enforcing the rules of chess.
AI Opponent
- Minimax Algorithm: A decision-making algorithm that evaluates the best move by simulating all possible moves and their outcomes.
- Alpha-Beta Pruning: An optimization for Minimax that reduces the number of nodes evaluated in the search tree, improving performance.
Move Evaluation
- Evaluation function that scores positions based on material, piece positions, and other strategic factors.
- Heuristic functions to prioritize moves that lead to advantageous positions (e.g., controlling the center, piece activity).
Game Flow and Player Interaction
- A turn-based system where the player alternates with the AI.
- Player input is captured via keyboard/mouse to select pieces and make moves.
GUI (Graphical User Interface)
- Chessboard and pieces are drawn using libraries like SFML, SDL, or Qt.
- Displays move history, captured pieces, and check/checkmate status.
Move History and Undo
- Keeps track of all moves for the current game session.
- Allows players to undo moves during gameplay.
Endgame Detection
- Checks for checkmate, stalemate, or draw conditions after each move.
- Alerts the player when the game is over.
Technologies Used
- C++
- Chess-specific libraries (optional, e.g., for move generation)
- SFML/SDL/Qt (for GUI rendering)
- Standard C++ libraries (STL for containers, vectors, etc.)
Learning Outcomes
- Deep understanding of game tree search algorithms (Minimax, Alpha-Beta Pruning)
- Experience in implementing a complex board game logic and AI
- Familiarity with chess strategy and move evaluation
- Skill in creating a basic GUI for interactive games
- Optimization techniques for AI performance
Possible Enhancements
- Implement different AI difficulty levels by adjusting the depth of the Minimax search or the evaluation function.
- Add features like hint systems, move suggestions, or an opening book for the AI.
- Include multiplayer functionality (local or online) for player vs player.
- Add a more advanced AI algorithm, such as Monte Carlo Tree Search (MCTS), for even stronger opponents.
- Create a more sophisticated GUI with animations for piece movements and checkmate sequences.